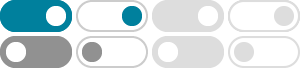
How to iterate any Map in Java - GeeksforGeeks
Jan 3, 2025 · In Java 8, you can iterate a map using Map.forEach (action) method and using lambda expression. This technique is clean and fast. Output : 5. Iterating over keys and searching for values (inefficient)
How to Iterate HashMap in Java? - GeeksforGeeks
Oct 16, 2021 · There is a numerous number of ways to iterate over HashMap of which 5 are listed as below: Iterate through a HashMap EntrySet using Iterators. Iterate through HashMap KeySet using Iterator. Iterate HashMap using for-each loop. Iterating through a HashMap using Lambda Expressions. Loop through a HashMap using Stream API.
dictionary - Iterating over Typescript Map - Stack Overflow
Jun 8, 2016 · If you have a Map of types (key, array) then you initialise the array this way: public cityShop: Map<string, Shop[]> = new Map(); And to iterate over it, you create an array from key values. Just use it as an array as in: keys = Array.from(this.cityShop.keys()); Then, in HTML, you can use: *ngFor="let key of keys"
c++ - How do you loop through a std::map? - Stack Overflow
I want to iterate through each element in the map<string, int> without knowing any of its string-int values or keys. What I have so far: void output(map<string, int> table) { map<string, int>::iterator it; for (it = table.begin(); it != table.end(); it++) { //How do I access each element?
Iterate Over a Map in Java - Baeldung
Dec 16, 2024 · In this tutorial, we’ll look at the different ways of iterating through the entries of a Map in Java and write a benchmark test to determine the most efficient method. Simply put, we can extract the contents of a Map using entrySet (), keySet (), or values (). Since these are all sets, similar iteration principles apply to all of them.
javascript - How to iterate a Map () object? - Stack Overflow
Feb 4, 2019 · Maps provide three ways to get iterators for their contents: keys - Iterates the keys in the map; values - Iterates the values; entries - Iterates the key and values, giving you [key, value] arrays (this is the default) As Nina notes, Maps also provide forEach, which loops through their contents giving the callback the value, key, and map as ...
How to iterate Map in Java - Tpoint Tech
How to iterate Map in Java. In Java, iteration over Map can be done in various ways. Remember that we cannot iterate over map directly using iterators, because Map interface is not the part of Collection. All maps in Java implements Map interface. There are following types of maps in Java: HashMap; TreeMap; LinkedHashMap
How to Iterate Through Map in C++ - Delft Stack
Feb 2, 2024 · Efficiently traversing the elements within a map is a fundamental skill for C++ programmers, and this article will serve as your compass through the various methods available for map iteration. To iterate over the elements of an …
Iterate through Map in C++: 6 New Methods (with Codes)
Nov 15, 2023 · In this article, we explored various methods to iterate through a map, including range-based for loops, explicit iterators, STL algorithms, and lambda functions. We also discussed best practices, handling map modifications, and additional tips and tricks to enhance the map iteration process.
Map.prototype[Symbol.iterator]() - JavaScript | MDN - MDN Web Docs
Feb 11, 2025 · The [Symbol.iterator]() method of Map instances implements the iterable protocol and allows Map objects to be consumed by most syntaxes expecting iterables, such as the spread syntax and for...of loops. It returns a map iterator object that yields the key-value pairs of the map in insertion order.