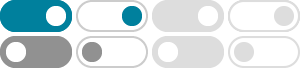
How does strtok () split the string into tokens in C?
Jul 15, 2016 · Note that strtok remembers its state for the tokenizing session. And for this reason it is not reentrant or thread safe (you should be using strtok_r instead). Another thing to know is that it actually modifies the original string. It writes '\0' for teh delimiters that it finds. One way to invoke strtok, succintly, is as follows:
Using strtok in c - Stack Overflow
Nov 12, 2011 · I need to use strtok to read in a first and last name and seperate it. How can I store the names where I can use them idependently in two seperate char arrays? #include <stdio.h> #include <
How does the strtok function in C work? - Stack Overflow
The important corollary is that you cannot use strtok on a const char* "hello world"; type of string, since you will get an access violation when you modify contents of a const char* string. The "good" thing about strtok is that it doesn't actually copy strings - so you don't need to manage additional memory allocation etc. But unless you ...
c - How to use strtok () - Stack Overflow
Sep 21, 2013 · Revised requirement. I would like to add a printf() statement outside the while loop and print '&' outside.I need it since I want to compare it later with another variable in the program.
What are the differences between strtok and strsep in C
Aug 28, 2011 · # ./example1_strtok Original String: aaa-bbb --ccc-ddd aaa bbb ccc ddd Original String: aaa In the output, you can see the token "bbb" and "ccc" one after another. strtok() does not indicate the occurrence of contiguous delimiter characters. Also, the strtok() modify the input string. Contiguous delimiter characters handling by strsep():
c++ - Using strtok with a std::string - Stack Overflow
NOTE: strtok may not be suitable in all situation as the string passed to function gets modified by being broken into smaller strings. Pls., ref to get better understanding of strtok functionality. How strtok works. Added few print statement to better understand the changes happning to string in each call to strtok and how it returns token.
Split string with multiple delimiters using strtok in C
Be warned about the general shortcomings of strtok() (from manual): These functions modify their first argument. These functions cannot be used on constant strings. The identity of the delimiting byte is lost. The strtok() function uses a static buffer while parsing, so it's not thread safe. Use strtok_r() if this matters to you.
What's the difference between strtok and strtok_r in C?
Mar 5, 2014 · The strtok_r() function is a reentrant version of strtok(). The context pointer last must be provided on each call. The context pointer last must be provided on each call. The strtok_r() function may also be used to nest two parsing loops within one another, as long as separate context pointers are used.
c - strtok - char array versus char pointer - Stack Overflow
Nov 3, 2010 · Possible Duplicate: strtok wont accept: char *str When using the strtok function, using a char * instead of a char [] results in a segmentation fault. This runs properly: char string[] = "hello
Do I need to free the strtok resulting string? - Stack Overflow
As others mentioned, strtok uses its first parameter, your input string, as the memory buffer. It doesn't allocate anything. It's stateful and non-thread safe; if strtok's first argument is null, it reuses the previously-provided buffer. During a call, strtok destroys the string, adding nulls into it and returning pointers to the tokens.