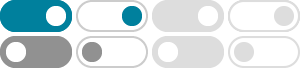
What does the ^ operator do in Java? - Stack Overflow
Jan 2, 2010 · Exponentiation in Java. As for integer exponentiation, unfortunately Java does not have such an operator. You can use double Math.pow(double, double) (casting the result to int if necessary). You can also use the traditional bit-shifting trick to compute some powers of two. That is, (1L << k) is two to the k-th power for k=0..63. See also
Combinatoric 'N choose R' in java math? - Stack Overflow
Feb 4, 2010 · binomialCoefficient, in Commons Math Returns an exact representation of the Binomial Coefficient, "n choose k", the number of k-element subsets that can be selected from an n-element set. Share
round up to 2 decimal places in java? - Stack Overflow
Jul 28, 2012 · I know this is 2 year old question but as every body faces a problem to round off the values at some point of time.I would like to share a different way which can give us rounded values to any scale by using BigDecimal class .Here we can avoid extra steps which are required to get the final value if we use DecimalFormat("0.00") or using Math.round(a * 100) / 100.
java - How to find GCD, LCM on a set of numbers - Stack Overflow
Jun 29, 2016 · I've used Euclid's algorithm to find the greatest common divisor of two numbers; it can be iterated to obtain the GCD of a larger set of numbers.
integer - How to implement infinity in Java? - Stack Overflow
Does Java have anything to represent infinity for every numerical data type? How is it implemented such that I can do mathematical operations with it? E.g. int myInf = infinity; //However it is done myInf + 5; //returns infinity myInf*(-1); //returns negative infinity I have tried using very large numbers, but I want a proper, easy solution.
How do I generate random integers within a specific range in Java ...
The Math.Random class in Java is 0-based. So, if you write something like this: Random rand = new Random(); int x = rand.nextInt(10); x will be between 0-9 inclusive. So, given the following array of 25 items, the code to generate a random number between 0 (the base of the array) and array.length would be:
java - Math.random () explanation - Stack Overflow
Nov 1, 2011 · This is a pretty simple Java (though probably applicable to all programming) question: Math.random() returns a number between zero and one. If I want to return an integer between zero and hundred, I would do: (int) Math.floor(Math.random() * 101) Between one and hundred, I would do: (int) Math.ceil(Math.random() * 100)
pow - Does Java have an exponential operator? - Stack Overflow
Is there an exponential operator in Java? For example, if a user is prompted to enter two numbers and they enter 3 and 2, the correct answer would be 9. import java.util.Scanner; public class
java - How to use comparison operators like >, =, < on BigDecimal ...
Jan 8, 2016 · Every object of the Class BigDecimal has a method compareTo you can use to compare it to another BigDecimal. The result of compareTo is then compared > 0, == 0 or < 0 depending on what you need.
java - How to calculate mean, median, mode and range from a set …
Nov 16, 2010 · ADDITION. If you are using Java 8 or higher, you can also determine the modes like this: public static List<Integer> getModes(final List<Integer> numbers) { final Map ...